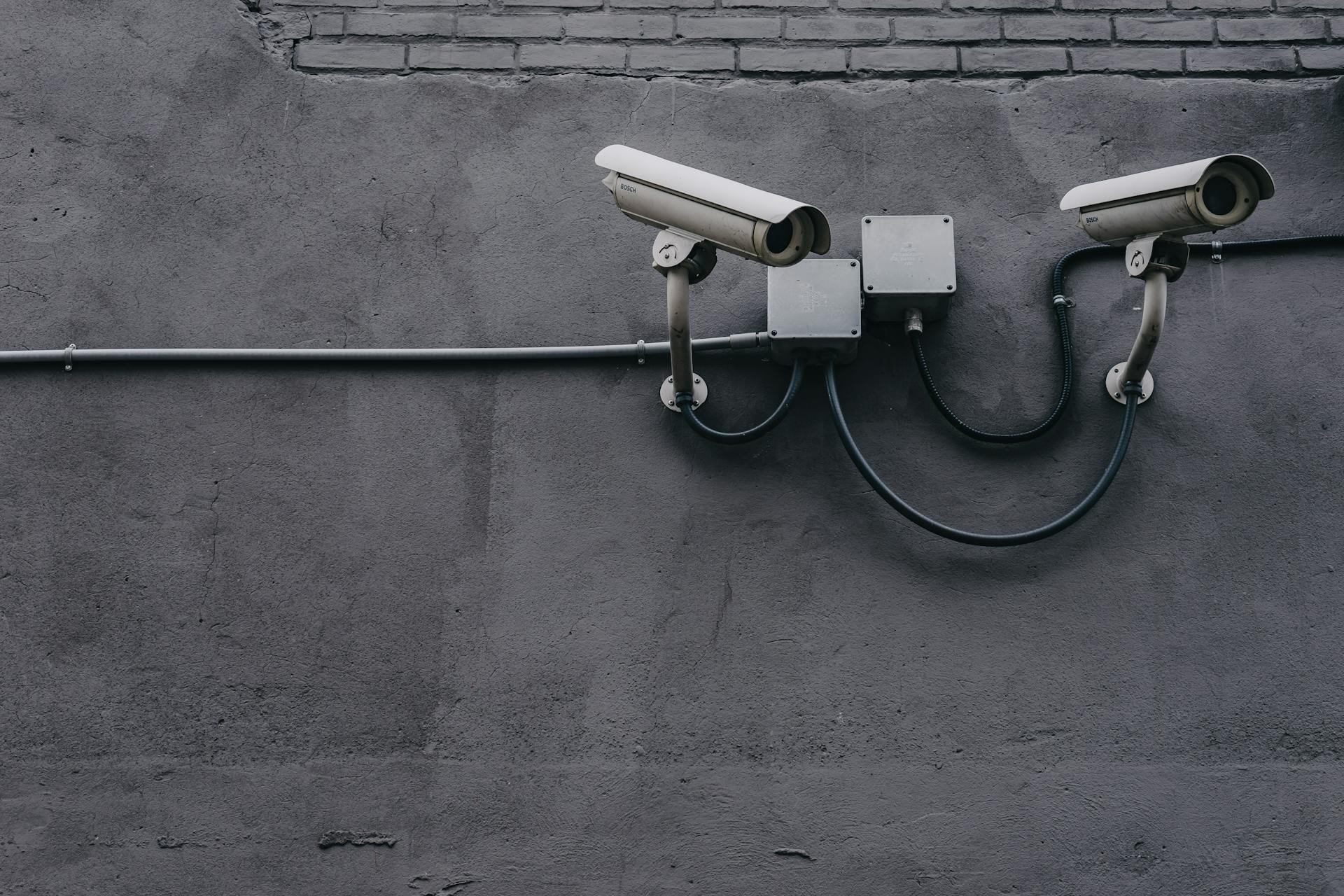
Spring Security Beginners Guide
Spring Security is a powerful and highly customizable authentication framework that provides support for various authentication methods, including basic authentication, form-based authentication, OAuth 2.0, OpenID Connect, and more. It also includes built-in support for authorization, which allows you to control access to resources based on user roles or permissions.
In this guide, we will explore the basic components of Spring Security and how to configure it to suit your needs.
1. Security Filter Chain
The Security Filter Chain is an important component of Spring Security that controls the flow of requests through the authentication, authorization, and exception handling filters. Think of it as a pipeline that processes incoming HTTP requests. When a request comes in, Spring security intercepts the request and passes it throught the filter chain before sending it to the DispatchServelet to ensure only authorized users access the resources.
The Security Filter Chain consists of three main components:
- Authentication Filters - These filters are responsible for authenticating users based on their credentials.
- Authorization Filters - These filters are responsible for authorizing requests based on user roles and permissions.
- Security Context Persistence Filter - Manages the SecurityContext and restores the security context for the request.
- Exception Translation Filter - These filters are responsible for handling exceptions that occur during authentication or authorization.
Spring Security provides a number of built-in authentication and authorization filters, such as Basic Authentication, Form Authentication, OAuth 2.0, OpenID Connect, and more. You can also create custom authentication and authorization filters to suit your specific needs. Security Filters are configured in the WebSecurityConfigurerAdapter
class.
By default when you start a new Spring Boot project with Spring Security, it includes SecurityAutoConfiguration that configures the Security Filter Chain for you. You can customize this configuration by overriding the configure(HttpSecurity http)
method of WebSecurityConfigurerAdapter which we will explore in more detail later on.
2. Authentication
Based on the configuration of Spring Security, if authentication is required, Spring Security need to check whether the user has provides valid credentials. Spring Security uses an Authentication Manager to authenticate users based on their credentials. The Authentication Manager manages the entire authentication process by delegating authentication requests to one or more Authentication Providers. The Authentication Manager iterates over a list of Authentication Providers to find one that supports the given authentication type.
ProviderManager is the default implementation of Authentication Manager. It takes a list of Authentication Provider as input and delegates authentication requests to these providers one by one until it finds a successful authentication or reaches the end of the filter chain.If none of them are able to authenticate, then Spring Security throws an AuthenticationException.
Authentication Provider uses a UserDetailsService to retrieve user details from the database based on their credentials. If the user is found in the database and matches with the provided credentials, then it returns an Authentication object that contains the user's information. Otherwise, it returns null.
After successful authentication, Spring Security stores the Authentication object in the SecurityContextHolder. The SecurityContext holds information about the current user's authentication status.
3. Authorization
Once authentication is successful, Spring Security needs to check whether the authenticated user has the necessary authorities (roles/permissions) to access the requested resource. Spring Security uses the authorities (granted roles/permissions) from the Authentication object to make authorization decisions. If the user does not have any of these authorities then it throws an AccessDeniedException.
4. Security Context Holder and Security Context
The SecurityContextHolder uses a thread-local variable to store the SecurityContext, which makes the Authentication object accessible during the entire lifecycle of a request in the same thread. When the user is authenticated, Spring Security places the Authentication object into the SecurityContext, making it accessible across the current request or session.
When the request completes and the response is sent back to the client, the SecurityContextHolder removes the Authentication object from its internal ThreadLocal variable. This makes the Authentication object unavailable for use during the next request in this thread.
Conclusion
Thats a lot to digest in one go and may be confusing as well for someone new to Spring Security. There a lot of moving parts in the whole process and I will try to summarise the above points here.
Security Filter Chain - a list of filters that are used by Spring Security to authenticate, authorize, and filter requests. Can be configured using XML or Java configuration to adapt to different security requirements.
Filter - A subcomponent of security filter chain which is responsible for authentication, authorization, or filtering based on the logic. Filters are invoked in the order they appear in the filter chain. Custom filters are added to implement your own logic like logging, auditing or custom authentication mechanisms (like JWT). For example, in JWT authentication, you would add a custom JWTAuthenticationFilter to validate the JWT token before granting access to secured endpoints.
Authentication Manager - coordinates multiple AuthenticationProvider implementations, ensuring that the application can support different types of authentication. For example, Spring Security supports JDBC and LDAP authentication.
Authentication Provider - handle the specific details of how users are authenticated by providing a mechanism for authenticating users using a particular authentication scheme (for example, username/password or token). Custom authentication providers may be added to support custom authentication schemes like JWT, SAML, OpenID Connect, etc.
User Details Service - is used to retrieve user information from the database and map it to a UserDetails object which is then passed on to AuthenticationManager for authentication. This separates the storage of user information (in the form of a database table) from the authentication logic.
As you can see, each of these components have their own role in the overall security flow which follows the separation of concerns principle. This makes it easier to understand and implement custom security mechanisms. In the next article we will look at how to implement custom AuthenticationProvider and UserDetailsService.